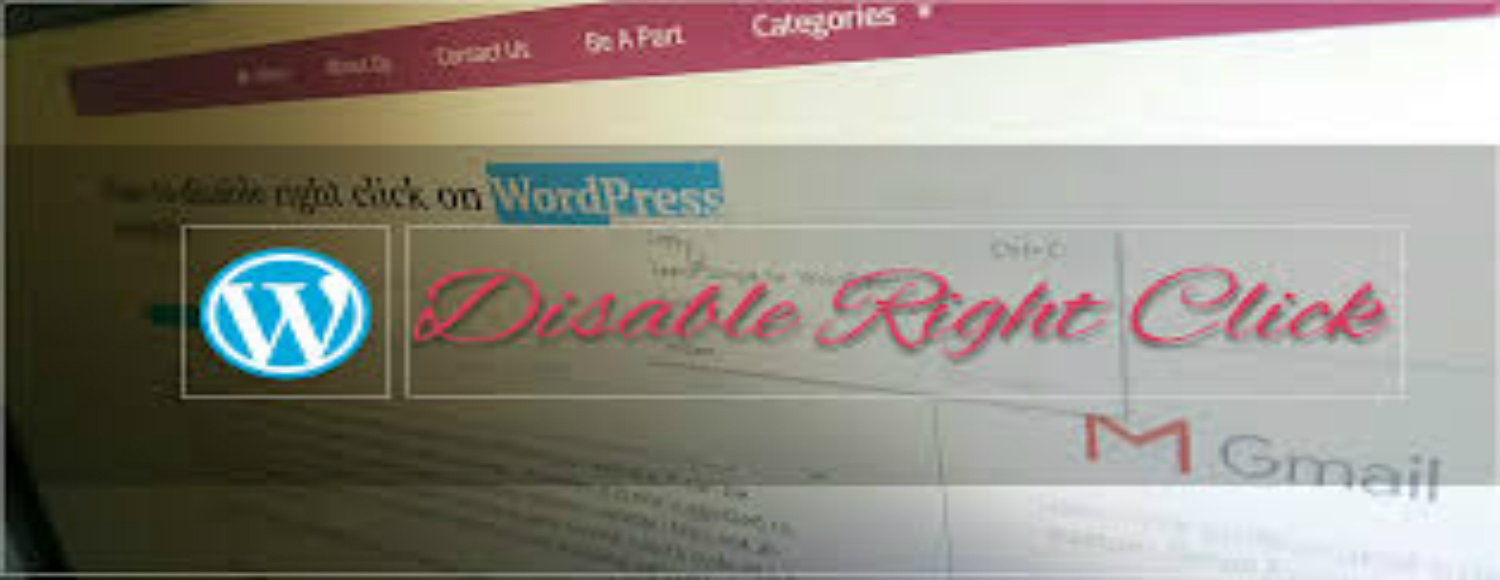
Disable right click on particular element like textbox, text area
Disabling right-click on specific elements like textboxes and text areas can prevent users from copying or pasting content, thereby enhancing data security and content protection. Here’s a simple way to do this using JavaScript:
Why Disable Right Click?
- Prevent Copying: To protect sensitive information or proprietary content.
- Enhance Security: To avoid unauthorized actions such as copying form values.
- Control User Interaction: To maintain the integrity of user input, especially in controlled environments.
How to Disable Right Click on Specific Elements
Here’s a step-by-step guide:
1. Using JavaScript
You can add an event listener to the specific element to disable the right-click context menu.
<!DOCTYPE html>
<html>
<head>
<title>Disable Right Click</title>
<script type=”text/javascript”>
document.addEventListener(‘DOMContentLoaded’, (event) => {
// Get the elements by their ID or class
const textBox = document.getElementById(‘myTextBox’);
const textArea = document.getElementById(‘myTextArea’);
// Disable right-click on the text box
textBox.addEventListener(‘contextmenu’, function(event) {
event.preventDefault();
});
// Disable right-click on the text area
textArea.addEventListener(‘contextmenu’, function(event) {
event.preventDefault();
});
});
</script>
</head>
<body>
<input type=”text” id=”myTextBox” placeholder=”Right-click disabled”>
<textarea id=”myTextArea” placeholder=”Right-click disabled”></textarea>
</body>
</html>
2. Using jQuery (Optional)
If you prefer jQuery, the process is quite similar:
<!DOCTYPE html>
<html>
<head>
<title>Disable Right Click</title>
<script src=”https://code.jquery.com/jquery-3.6.0.min.js”></script>
<script type=”text/javascript”>
$(document).ready(function() {
// Disable right-click on the text box
$(‘#myTextBox’).on(‘contextmenu’, function(event) {
event.preventDefault();
});
// Disable right-click on the text area
$(‘#myTextArea’).on(‘contextmenu’, function(event) {
event.preventDefault();
});
});
</script>
</head>
<body>
<input type=”text” id=”myTextBox” placeholder=”Right-click disabled”>
<textarea id=”myTextArea” placeholder=”Right-click disabled”></textarea>
</body>
</html>
Important Considerations
- User Experience: Disabling right-click might frustrate users who are accustomed to using it for spell check or other browser features.
- Bypass Methods: Users can still view the page source or use developer tools to access the content.
- Balance Security and Usability: Ensure that the security measures do not overly compromise user experience.
By following the steps above, you can effectively disable right click on specific elements while considering the potential impacts on user interaction.
Concept Infoway is a leading Microsoft Certified Offshore Development Company. In business since the year 2000, we serve as a one-stop offshore development center for all our clients’ IT needs. Whether it is Microsoft technology-based development, Open Source technology-based development, mobile app development, online marketing, quality assurance, or Managed IT Services (MITS), businesses can rely on us for the best and budget-friendly solutions.